Subscribe to the newsletter
Functional programming has emerged as a transformative paradigm in the world of software development, and its impact is particularly pronounced in the JavaScript ecosystem. Whether you’re a seasoned developer or just starting your coding journey, understanding the core concepts of functional programming can elevate your skills and enable you to write cleaner, more maintainable, and bug-free code.
In this blog, we’ll take a hands-on approach to unravel the essential concepts of functional programming using JavaScript as our medium.
What is functional programming?
Functional programming is a declarative approach to software development that centers around constructing applications through the composition of pure functions. It emphasizes steering clear of shared state and mutable data while relocating side effects to the program’s periphery. For a thorough understanding of functional programming, it is important to understand some key concepts, such as:
1. Declarative programming Vs. Imperative programming
Declarative programming and imperative programming are two distinct approaches to writing code in computer programming.
Imperative programming is the more traditional of the two. In this approach, the programmer provides explicit instructions to the computer, detailing step by step how a task should be accomplished. It relies heavily on statements, loops, and mutable variables to control the flow of execution. An example in JavaScript:
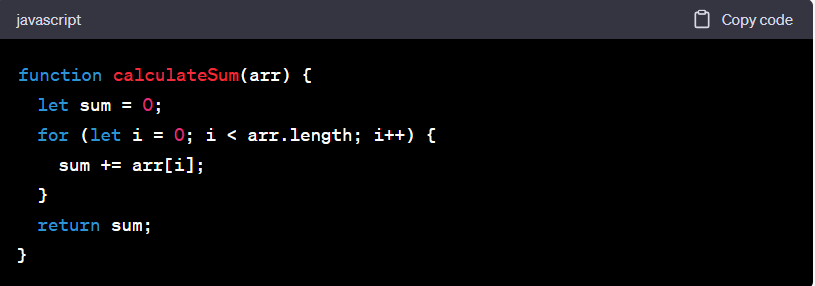
Here, we explicitly specify the process of iterating through an array and incrementally updating a variable to calculate the sum.
Declarative programming, on the other hand, focuses on expressing what should be done, rather than how to do it. The programmer describes the desired outcome or result, and the underlying system or framework takes care of the implementation details. It relies on high-level abstractions and functional constructs to simplify complex operations. An example in JavaScript:

In this declarative code, we use the reduce function to succinctly express the desired operation without detailing the iteration process.
In summary, declarative programming prioritizes a higher-level, more abstract approach, while imperative programming involves specifying explicit steps for task execution. The choice between them depends on the task at hand and the desired balance between control and abstraction in your code. As mentioned above, functional programming makes use of declarative programming.
2. Pure Functions
A pure function is a fundamental concept in functional programming. It is a type of function that adheres to specific principles, making it predictable, reliable, and easier to reason about. The key characteristics of a pure function are:
- Deterministic: A pure function produces the same output for the same set of input parameters every time it is called. It doesn’t rely on external factors, such as global variables or system state, which can introduce randomness or unpredictability.
- No side effects: A pure function has no side effects, meaning it doesn’t modify any external state or variables, interact with the outside world (e.g., file system or network), or perform any observable actions beyond computing and returning a value. It operates solely on its input parameters.
Here’s an example of a pure function in JavaScript:
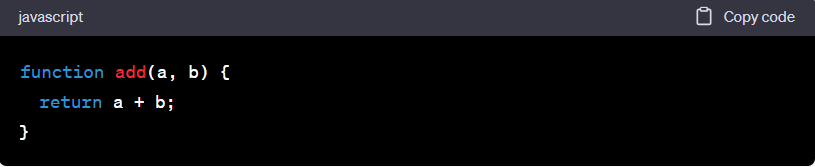
The add function is pure because it takes two arguments, a and b, and returns their sum. It doesn’t modify any global variables, interact with external resources, or have any side effects.
Pure functions consistently steer clear of side effects, while impure functions invariably introduce side effects. This relationship aligns with the following principles: immutable data is employed to prevent shared state, and the use of pure functions helps circumvent mutations. When seeking to hire Elixir developer, it’s crucial to prioritize candidates who understand and embrace these fundamental concepts, ensuring the development of robust and maintainable codebases.
3. Functions as First-Class Citizens in Functional Programming
In functional programming, functions are accorded a unique status: they are treated as “first-class citizens.” This distinction means that functions are not merely blocks of code executed within a program but are instead elevated to a level of importance and flexibility that allows them to be manipulated and utilized in ways akin to traditional data types.
This stands in contrast to the object-oriented programming paradigm, where classes typically hold the position of “first-class citizens.”
Here’s a closer look at what it means for functions to be first-class citizens in the realm of functional programming:
Functions as Values:
Functions can be assigned to variables just like any other data type. This means you can encapsulate functionality within a function and reference it by name, facilitating cleaner and more modular code. For example:

Functions as Arguments:
Functions can be passed as arguments to other functions. This concept allows for the creation of higher-order functions that operate on other functions, offering a powerful mechanism for abstraction and dynamic behaviour. For example:
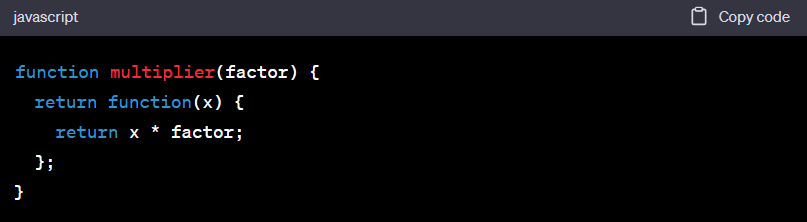
Functions as Return Values:
Functions can also be returned as values from other functions. This capability is particularly valuable for constructing closures, factory functions, or function generators, which produce specialized functions based on certain conditions or parameters. For example:

By treating functions as first-class citizens, functional programming encourages a style of coding that emphasizes composition, reusability, and abstraction, all while maintaining a clear focus on the behavior of functions themselves.
4. Function Composition
Function composition is a fundamental concept in functional programming that involves combining or chaining multiple functions together to create a new function. This new function represents the composition of the individual functions, with the output of one function serving as the input for the next.
Function composition allows you to build complex operations or transformations by breaking them down into smaller, reusable, and composable parts.
How functional composition can be used to process an order?
Functional composition streamlines order processing by breaking it into modular, reusable functions that can be combined into a pipeline. Each function handles specific tasks, like validation, calculations, or inventory updates.
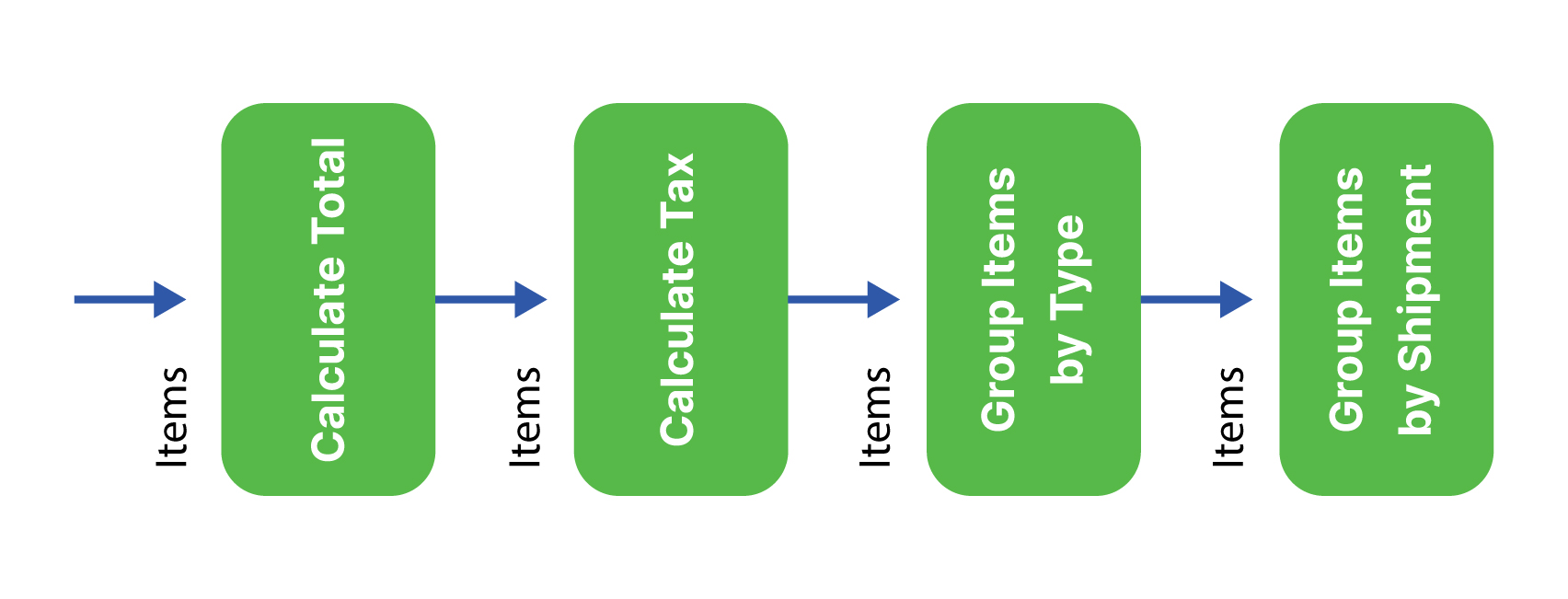
The composed functions create a seamless flow for processing orders, making code more readable and maintainable. Error handling can be integrated at each step, ensuring robust processing. Finally, the composed result represents the processed order, ready for further actions like sending confirmation emails or database updates.
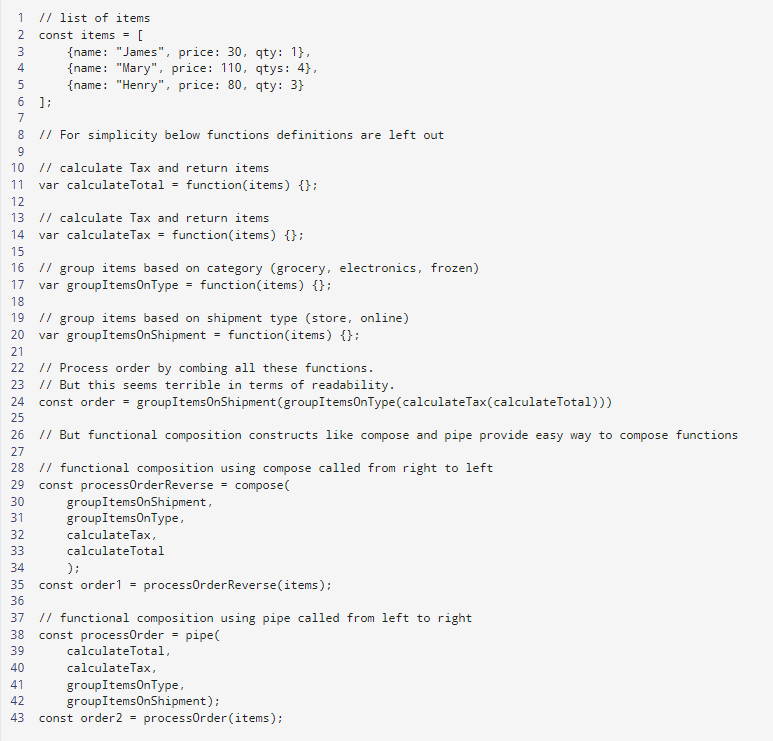
Conclusion
Functional programming stands as a pivotal paradigm in modern software development, emphasizing clean, predictable, and modular code. With JavaScript as our guide, we’ve delved into its essential concepts, from declarative programming to pure functions and function composition.
Confiz, armed with its extensive technological expertise can play a pivotal role in harnessing the power of functional programming for your software needs.
Our adept team with their vast experience in programming and software development can help you navigate the intricacies of functional programming, crafting robust and efficient solutions that streamline your development processes and elevate the quality of your software products.
So, contact us at marketing@confiz.com and allow our experts to help you learn more!